2022. 1. 6. 06:52ㆍJava/명품 Java
**모든 소스코드는 해당 문제가 수록된 장까지의 교재 내용만을 이용하여 작성하였습니다**
[4-1] 자바 클래스 작성 연습을 해보자. 다음 main( )메소드를 실행하였을 때 예시와 같이 출력되도록 TV 클래스를 작성하여라.
소스코드
class TV{
String company;
int year, inches;
public TV() {}
public TV(String company, int year, int inches) {
this.company = company;
this.year = year;
this.inches = inches;
}
public void show() {
System.out.println(company + "에서 만든 " + year + "년형 " + inches + "인치 TV");
}
}
public class Main{
public static void main(String[] args){
TV myTV = new TV("LG", 2017, 32);
myTV.show();
}
}
결과
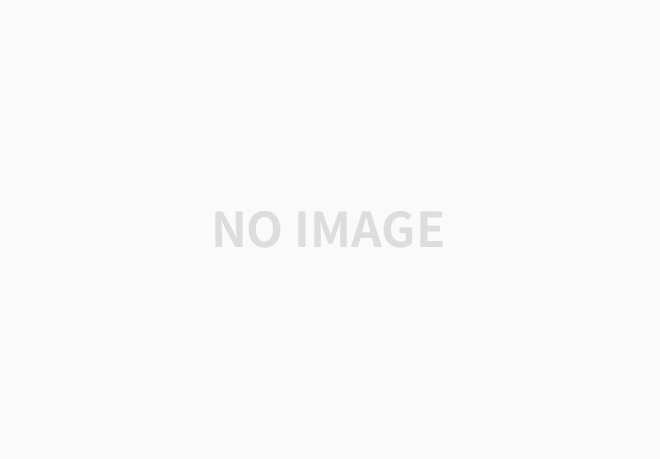
[4-2] Grade 클래스를 작성해보자. 3과목의 점수를 입력받아 Grade 객체를 생성하고 성적 평균을 출력하는 main()과 실행예시는 다음과 같다.
소스코드
import java.util.Scanner;
class Grade{
private int math, science, english;
public Grade() {}
public Grade(int math, int science, int english) {
this.math = math;
this.science = science;
this.english = english;
}
public int average() {
return (math+science+english)/3;
}
}
public class Main{
public static void main(String[] args){
Scanner scanner = new Scanner(System.in);
System.out.print("수학, 과학, 영어 순으로 3개의 점수 입력>>");
int math = scanner.nextInt();
int science = scanner.nextInt();
int english = scanner.nextInt();
Grade me = new Grade(math, science, english);
System.out.println("평균은 " + me.average());
scanner.close();
}
}
결과
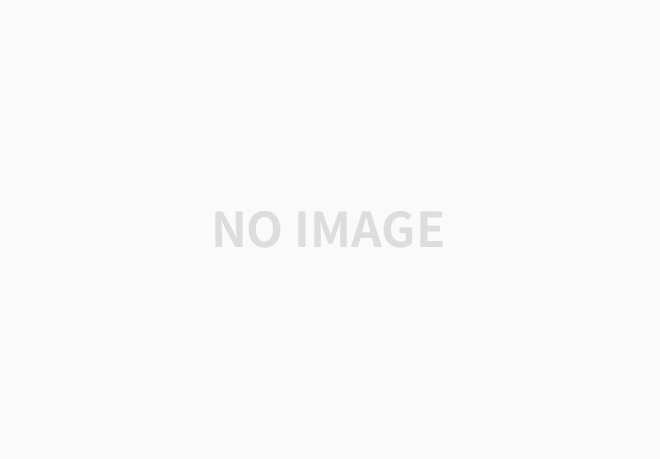
[4-3] 노래 한 곡을 나타내는 Song 클래스를 작성하라.
소스코드
class Song{
private int year;
private String title, artist, country;
public Song() {}
public Song(int year, String country, String artist, String title) {
this.year = year;
this.country = country;
this.artist = artist;
this.title = title;
}
public void show() {
System.out.println(year + "년 " + country + "국적의 " + artist +"가 부른 " + title);
}
}
public class Main{
public static void main(String[] args){
Song song = new Song(1987, "스웨덴", "ABBA", "Dancing Queen");
song.show();
}
}
결과
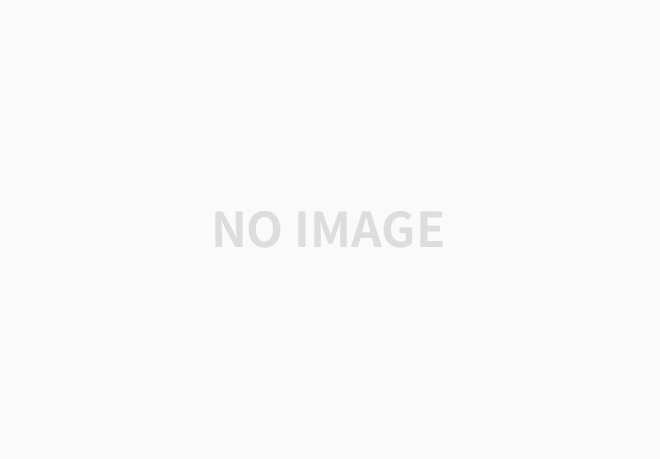
[4-4] 직사각형을 표현하는 Rectangle 클래스 작성
소스코드
class Rectangle{
private int x, y, width, height;
public Rectangle() {}
public Rectangle(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
public int square() {
return width*height;
}
public void show() {
System.out.println("(" + x + "," + y + ")에서 크기가 " + width + "x" + height + "인 사각형");
}
public boolean contains(Rectangle r) {
if((this.x < r.x && r.x+r.width < this.x+ this.width)&&(this.y<r.y && r.y+r.height < this.y+this.height)) {
return true;
}else {
return false;
}
}
}
public class Main{
public static void main(String[] args){
Rectangle r = new Rectangle(2,2,8,7);
Rectangle s = new Rectangle(5,5,6,6);
Rectangle t = new Rectangle(1,1,10,10);
r.show();
System.out.println("s의 면적은 " + s.square());
if(t.contains(r)) System.out.println("t는 r을 포함합니다.");
if(t.contains(s)) System.out.println("t는 s를 포함합니다.");
}
}
결과
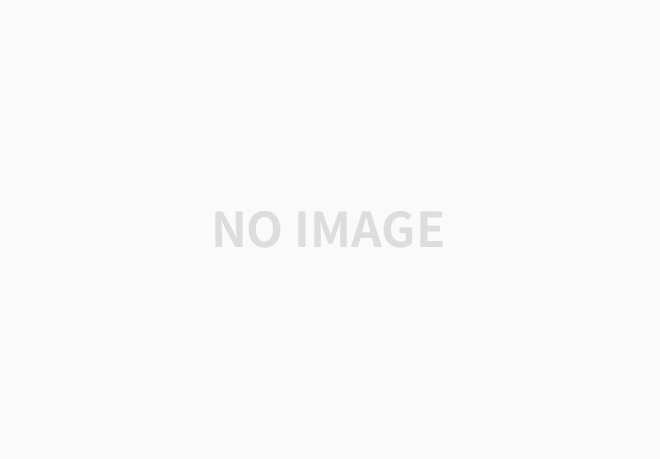
[4-5] Circle 클래스와 CircleManager 클래스를 완성하라.
소스코드
import java.util.Scanner;
class Circle{
private double x,y;
private int radius;
public Circle(double x, double y, int radius) {
this.x = x; this.y = y; this.radius = radius;
}
public void show() {
System.out.println("(" + x + "," + y + ")" + radius);
}
}
public class CircleManager{
public static void main(String[] args){
Scanner scanner = new Scanner(System.in);
Circle c[] = new Circle[3];
for(int i = 0; i<c.length; i++) {
System.out.print("x, y, radius >>");
double x = scanner.nextDouble();
double y = scanner.nextDouble();
int radius = scanner.nextInt();
c[i] = new Circle(x, y, radius);
}
for(int i = 0; i<c.length; i++) c[i].show();
scanner.close();
}
}
결과
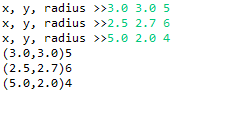
[4-6] 5번의 Circle 클래스와 CircleManager 클래스 수정하기
소스코드
import java.util.*;
class Circle{
double x,y;
int radius;
public Circle(double x, double y, int radius) {
this.x = x; this.y = y; this.radius = radius;
}
public String show() {
return ("(" + x + "," + y + ")" + radius);
}
}
public class CircleManager{
public static void main(String[] args){
Scanner scanner = new Scanner(System.in);
Circle c[] = new Circle[3];
for(int i = 0; i<c.length; i++) {
System.out.print("x, y, radius >>");
double x = scanner.nextDouble();
double y = scanner.nextDouble();
int radius = scanner.nextInt();
c[i] = new Circle(x, y, radius);
}
int max = 0;
Circle maxCircle = null ;
for(int i = 0; i<c.length; i++) {
if(c[i].radius >max) {
max = c[i].radius;
maxCircle = c[i];
}
}
System.out.println("가장 면적이 큰 원은 " + maxCircle.show());
scanner.close();
}
}
결과
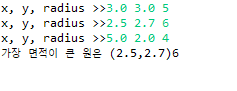
[4-7] 한 달의 할 일을 표현하는 MonthSchedule 클래스를 작성하라.
소스코드
import java.util.Scanner;
class Day{
private String work;
public void set(String work) {this.work = work;}
public String get() {return work;}
public void show() {
if(work == null) System.out.println("없습니다.");
else System.out.println(work + "입니다.");
}
}
public class MonthSchedule{
Scanner scanner = new Scanner(System.in);
Day[] days;
public MonthSchedule(int day){
days = new Day[day];
for(int i=0; i<days.length; i++)
days[i] = new Day();
}
public void input() {
System.out.print("날짜(1~30)?");
int day = scanner.nextInt();
System.out.print("할일(빈칸없이입력)?");
String schedule = scanner.next();
days[day-1].set(schedule);
}
public void view() {
System.out.print("날짜(1~30)?");
int day = scanner.nextInt();
System.out.print(day + "일의 할 일은 ");
days[day-1].show();
}
public void finish() {
System.out.println("프로그램을 종료합니다.");
}
public void run() {
System.out.println("이번달 스케쥴 관리 프로그램.");
while(true) {
System.out.print("할일(입력:1, 보기:2, 끝내기:3) >>");
int n = scanner.nextInt();
if(n == 1) {
input();
}else if(n == 2) {
view();
}else {
finish();
break;
}
System.out.println();
}
}
public static void main(String[] args){
MonthSchedule april = new MonthSchedule(30);
april.run();
}
}
결과
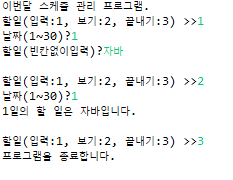
[4-8] 이름(name), 전화번호(tel) 필드와 생성자 등을 가진 Phone 클래스를 작성하고, 실행 예시와 같이 작동하는 PhoneBook 클래스를 작성하라.
소스코드
import java.util.Scanner;
class Phone{
private String name;
private String tel;
public Phone(){}
public Phone(String name, String tel){this.name = name; this.tel = tel;}
public String getName() {return name;}
public String getTel() {return tel;}
}
public class PhoneBook{
int n;
Scanner sc = new Scanner(System.in);
Phone[] phonebook;
public void run() {
System.out.print("인원수>>");
n = sc.nextInt();
phonebook = new Phone[n];
for(int i = 0; i<n; i++) {
phonebook[i] = new Phone();
}
this.input();
this.search();
}
public void input() {
for(int i = 0; i<n;i++) {
System.out.print("이름과 전화번호(이름과 번호는 빈 칸없이 입력)>>");
String name = sc.next();
String tel = sc.next();
phonebook[i] = new Phone(name, tel);
}
System.out.println("저장되었습니다...");
}
public void search() {
String searchname;
int index = n;
while(true){
System.out.print("검색할 이름>>");
searchname = sc.next();
if(searchname.equals("그만"))
break;
for(int i = 0; i<n; i++) {
if(searchname.equals(phonebook[i].getName()))
index = i;
}
if(index !=n)
System.out.println(searchname + "의 번호는 " + phonebook[index].getTel() + " 입니다.");
else
System.out.println(searchname + " 이 없습니다.");
}
}
public static void main(String[] args){
PhoneBook pb = new PhoneBook();
pb.run();
}
}
결과
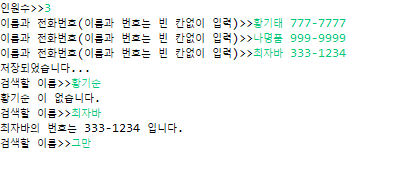
[4-9] 다음 2개의 static 가진 ArrayUtil 클래스를 만들어보자. 다음 코드의 실행 결과를 참고하여 concat( )와 print( )를 작성하여 ArrayUtil 클래스를 완성하라.
소스코드
class ArrayUtil{
public static int[] concat(int[] a, int[] b) {
int[] c= new int[a.length+b.length];
for(int i = 0; i<a.length; i++)
c[i] = a[i];
for(int i = a.length; i<a.length+b.length; i++)
c[i] = b[i-a.length];
return c;
}
public static void print(int[] a) {
System.out.print("[ ");
for(int i = 0; i<a.length; i++)
System.out.print(a[i] + " ");
System.out.print("]");
}
}
public class StaticEx{
public static void main(String[] args){
int[] array1 = {1, 5, 7, 9};
int[] array2 = {3, 6, -1, 100, 77};
int[] array3 = ArrayUtil.concat(array1, array2);
ArrayUtil.print(array3);
}
}
결과

[4-10] 다음과 같은 Dictionary 클래스가 있다. 실행 결과와 같이 작동하도록 Dictionary 클래스의 kor2Eng( ) 메소드와 DicApp 클래스를 작성하라.
소스코드
import java.util.*;
class Dictionary{
private static String[] kor = {"사랑", "아기", "돈", "미래", "희망"};
private static String[] eng = {"love", "baby", "money", "future", "hope"};
public static String kor2Eng(String word) {
for(int i = 0; i<kor.length; i++) {
if(word.equals(kor[i]))
return(word + "은 " + eng[i]);
}
return(word + "는 저의 사전에 없습니다.");
}
}
public class DicApp{
Scanner sc = new Scanner(System.in);
String searchWord;
public void Run() {
System.out.println("한영 단어 검색 프로그램입니다.");
while(true) {
System.out.print("한글 단어?");
searchWord = sc.next();
if(searchWord.equals("그만"))
break;
System.out.println(Dictionary.kor2Eng(searchWord));
}
}
public static void main(String[] args){
DicApp dic = new DicApp();
dic.Run();
}
}
결과
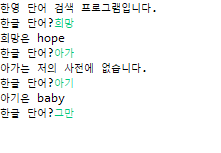
[4-11] 다수의 클래스를 만들고 활용하는 연습을 해보자. 더하기(+), 빼기(-), 곱하기(*), 나누기(/)를 수행하는 각 클래스 Add, Sub, Mul, Div를 만들어라. 이들은 모두 다음 필드와 메소드를 가진다.
-int 타입의 a,b 필드: 2개의 피연산자
-void setValue(int a, int b): 피연산자 값을 객체 내에 저장한다.
-int calculate( ) : 클래스의 목적에 맞는 연산을 실행하고 결과를 리턴한다.
main( ) 메소드에서는 다음 실행 사례와 같이 두 정수와 연산자를 입력받고 Add, Sub, Mul, Div 중에서 이 연산을 실행할 수 있는 객체를 생성하고 setValue( )와 calculate( )를 호출하여 결과를 출력하도록 작성하라.
소스코드
import java.util.*;
class Add{
private int a, b;
public void setValue(int a, int b) {this.a = a; this.b = b;}
public int calculate() {return a+b;}
}
class Sub{
private int a, b;
public void setValue(int a, int b) {this.a = a; this.b = b;}
public int calculate() {return a-b;}
}
class Mul{
private int a, b;
public void setValue(int a, int b) {this.a = a; this.b = b;}
public int calculate() {return a*b;}
}
class Div{
private int a, b;
public void setValue(int a, int b) {this.a = a; this.b = b;}
public int calculate() {return a/b;}
}
public class Calculate{
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.print("두 정수와 연산자를 입력하시오>>");
int a = sc.nextInt();
int b = sc.nextInt();
String op = sc.next();
switch(op) {
case "+":
Add add = new Add();
add.setValue(a,b);
System.out.println(add.calculate());
break;
case "-":
Sub sub = new Sub();
sub.setValue(a,b);
System.out.println(sub.calculate());
break;
case "*":
Mul mul = new Mul();
mul.setValue(a,b);
System.out.println(mul.calculate());
break;
case "/":
Div div = new Div();
div.setValue(a,b);
System.out.println(div.calculate());
break;
default:
System.out.println("올바르지 않은 연산자입니다.");
}
}
}
결과
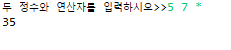
[4-12] 간단한 콘서트 예약 시스템을 만들어보자. 예약 시스템의 기능은 다음과 같다.
-공연은 하루에 한 번 있다.
-좌석은 S석, A석, B석으로 나뉘며, 각각 10개의 좌석이 있다.
-예약 시스템의 메뉴는 "예약", "조회", "취소", "끝내기"가 있다.
-예약은 한 자리만 가능하고, 좌석 타입, 예약자 이름, 좌석 번호를 순서대로 입력받아 예약한다.
-조회는 모든 좌석을 출력한다.
-취소는 예약자의 이름을 입력받아 취소한다.
-없는 이름, 없는 번호, 없는 메뉴, 잘못된 취소 등에 대해서 오류 메세지를 출력하고 사용자가 다시 시도하도록 한다.
소스코드
import java.util.*;
class Menu{
static Scanner sc2 = new Scanner(System.in);
static String[][] Seats = new String[3][10];
static int seat, seatNum, cancelNum;
static String name, cancelName;
static void Set() {
for(int i = 0; i<Seats.length; i++) {
for(int j = 0; j<Seats[i].length; j++)
Seats[i][j] = "---";
}
}
static void getSeatType(int seat) {
if(seat == 1)
System.out.print("S>> ");
else if(seat ==2)
System.out.print("A>> ");
else if(seat ==3)
System.out.print("B>> ");
}
static void Reservation() {
while(true) {
System.out.print("좌석구분 S(1), A(2), B(3)>>");
seat = sc2.nextInt();
if(seat<1 || seat>3)
System.out.println("잘못된 좌석 타입입니다. 다시 시도하세요.");
else {
getSeatType(seat);
break;
}
}
while(true) {
for(int i = 0; i<Seats[0].length; i++)
System.out.print(Seats[seat-1][i] + " ");
System.out.println();
System.out.print("이름>>");
name = sc2.next();
System.out.print("번호>>");
seatNum = sc2.nextInt();
if(seatNum < 1 || seatNum >10 ||!(Seats[seat][seatNum-1].equals("---"))){
System.out.println("예약할 수 없는 좌석입니다. 다시 시도하세요.");
}else {
Seats[seat-1][seatNum-1] = name;
break;
}
}
}
static void getInfo() {
for(int i =0; i<Seats.length; i++) {
getSeatType(i+1);
for(int j= 0; j<Seats[i].length; j++)
System.out.print(Seats[i][j] + " ");
System.out.println();
}
System.out.println("<<<조회를 완료하였습니다.>>>");
}
static void Cancel() {
while(true) {
System.out.print("좌석 S:1, A:2, B:3>>");
cancelNum = sc2.nextInt();
if(cancelNum<1 || cancelNum>3)
System.out.println("잘못된 좌석 타입입니다. 다시 시도하세요.");
else
break;
}
while(true) {
getSeatType(cancelNum);
for(int i =0; i<Seats[cancelNum-1].length; i++)
System.out.print(Seats[cancelNum-1][i] + " ");
System.out.println();
System.out.print("이름>>");
cancelName = sc2.next();
boolean isNameThere = false;
for(int i= 0; i<Seats[cancelNum-1].length; i++) {
if(cancelName.equals(Seats[cancelNum-1][i])) {
Seats[cancelNum-1][i] = "---";
isNameThere = true;
}
}
if(isNameThere == false)
System.out.println("예약자가 아닙니다. 다시 시도하세요.");
else
break;
}
}
}
public class ReservSystem{
Scanner sc = new Scanner(System.in);
public void Run() {
System.out.println("명품콘서트홀 예약 시스템입니다.");
Menu.Set();
while(true) {
System.out.print("예약:1, 조회:2, 취소:3, 끝내기:4>>");
int menu = sc.nextInt();
if(menu == 4) break;
switch(menu) {
case 1: Menu.Reservation(); break;
case 2: Menu.getInfo(); break;
case 3: Menu.Cancel(); break;
default: System.out.println("잘못된 메뉴입니다. 다시 입력하세요."); break;
}
}
}
public static void main(String[] args){
ReservSystem rev = new ReservSystem();
rev.Run();
}
}
결과
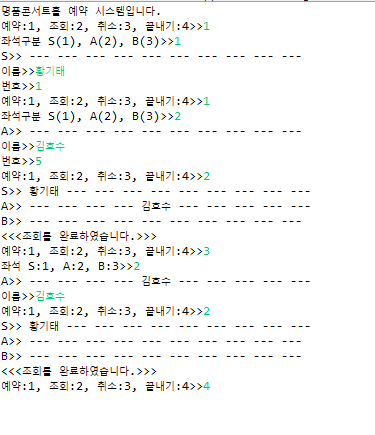
'Java > 명품 Java' 카테고리의 다른 글
[명품 Java Programming] 5장 Open Challenge (0) | 2022.01.07 |
---|---|
[명품 Java Programming] 4장 Open challenge (0) | 2022.01.05 |
[명품 Java Programming] 7장 Open Challenge (0) | 2021.12.07 |
[명품 Java Programming] 6장 Open Challenge (0) | 2021.12.06 |